Introduction
In this tutorial, we will be building a Bible app using Flask. We will be implementing an API available here. We will be implementing this application using requests library which will help us make HTTP requests.
Install modules
To begin this tutorial we will need a few libraries that are Flask as our web server and requests for making HTTP requests. To install open your terminal and run the commands below.
pip3 install Flask
pip3 install requests
File structure
Let’s create a folder called flask-bible, inside the folder create a folder called templates and insert a file called index.html.
Import modules
Inside the flask-bible folder create a file called app.py and add import the following modules as shown in the code below.
from flask import Flask ,render_template
import requests
Initialize app
Let’s now initialize our app as shown below.
app = Flask(__name__)
Create the view
Now let’s create a view that prompts the user to enter the book, chapter, and verse and then renders the content to the template created earlier.
@app.route('/',methods=['GET','POST'])
def index():
if request.method == "POST":
book = request.form['book']
chapter = request.form['chapter']
verse = request.form['verse']
url = f"https://bible-api.com/{book}%20{chapter}:{verse}"
response = requests.get(url).json()
reference = response['reference']
text = response['text']
return render_template("index.html",reference=reference, text=text)
return render_template("index.html")
if __name__ == "__main__":
app.run(debug=True)
Create a template
Let’s now create a template where users enter the book, chapter, and verse and then renders the content below the form. Inside the index.html form created above add the following.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<style>
.title {
text-align: center;
}
.prompt {
text-align: center;
}
.bible {
text-align: center;
}
</style>
<div class="title">
<h2>THE HOLY BIBLE</h2>
</div>
<form method="POST" class="prompt">
Book : <input type="text" name="book" required>
Chapter : <input type="number" name="chapter" required>
verse : <input type="number" name="verse" required>
<button type="submit">Submit</button>
</form>
<div class="bible">
<h4>{{reference}}</h4>
<p>{{ text }}</p>
</div>
</body>
</html>
Run
Let’s now run our application, to do so open your terminal and run the command below.
python3 app.py
Results
After you run the above command your application should be available at the address [ http:127.0.0.1:5000 ].
Open your browser at the address above and your app should be available as shown below.
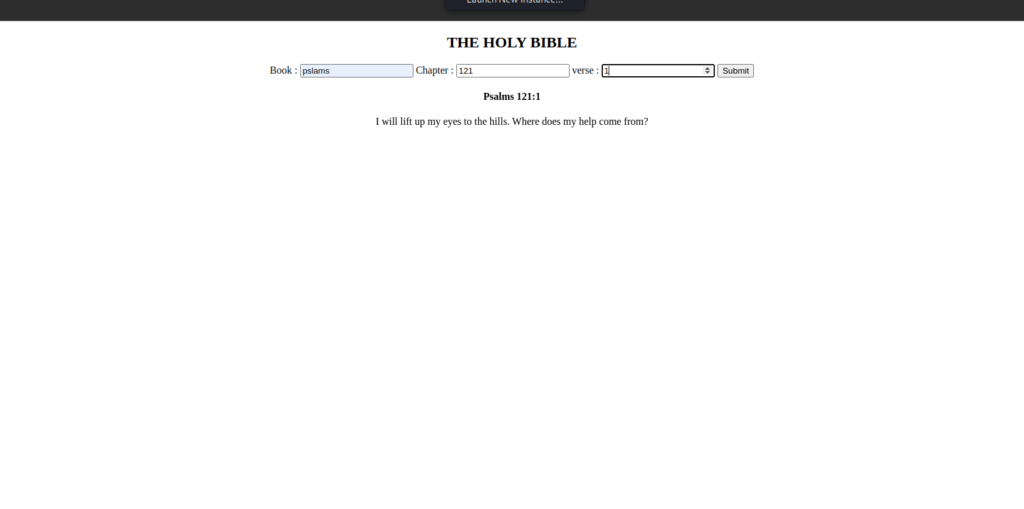
There you have it. Thanks for reading. Happy Coding.