Introduction
In this tutorial, we will be building a Flask app that converts an RGB value to a color name. This application will help in simplifying color identification. For this tutorial, we will be using Flask as our web server and webcolors as our RGB library.
Table of Contents
File Structure
To begin we will first need to create a few files and folders, Create a folder called rgb-app, inside it create a folder called templates and create a file called index.html. Now inside the rgb-app folder create a file called app.py
Install modules
To begin we will first need to install Flask and webcolors, to do so run the following commands below in your terminal.
pip3 install Flask
pip3 install webcolors
Create app
Inside the app.py import the following modules
from flask import Flask, render_template, request
import webcolors
Now let’s initialize our application as shown below.
app = Flask(__name__)
Next, let’s create a function to convert our RGB values.
def rgb_to_color_name(rgb):
# Convert RGB value to closest color name
try:
color_name = webcolors.rgb_to_name(rgb)
return color_name
except ValueError:
return None
SEE ALSO: How to Build a Flask Weather App Using Python
Now let’s create our route for the application as shown below.
@app.route('/', methods=['GET', 'POST'])
def color_converter():
if request.method == 'POST':
# Get RGB values from the form
r = int(request.form['r'])
g = int(request.form['g'])
b = int(request.form['b'])
rgb_value = (r, g, b)
color_name = rgb_to_color_name(rgb_value)
return render_template('index.html', color_name=color_name)
else:
return render_template('index.html')
if __name__ == '__main__':
app.run(debug=True)
The above route receives RGB values from the template and then converts them and then returns the color name to the template.
Create template
Inside the index.html file we created earlier insert the code below.
<!DOCTYPE html>
<html>
<head>
<title>RGB to Color Name Converter</title>
</head>
<body>
<style>
div {
text-align:center;
}
</style>
<div>
<h1>RGB to Color Name Converter</h1>
</div>
<div>
<form method="POST" action="/">
<label for="r">R:</label>
<input type="number" name="r" id="r" min="0" max="255" required><br><br>
<label for="g">G:</label>
<input type="number" name="g" id="g" min="0" max="255" required><br><br>
<label for="b">B:</label>
<input type="number" name="b" id="b" min="0" max="255" required><br><br>
<input type="submit" value="Convert">
</form>
</div>
<div>
{% if color_name %}
<h2>Color Name: {{ color_name }}</h2>
{% endif %}
</div>
</body>
</html>
Run
To run the app, open your terminal and enter the command below. After running the command below our application should be available at the address [ http://localhost:5000 ]
python3 app.py
Results
Here is a screenshot of the finished application.
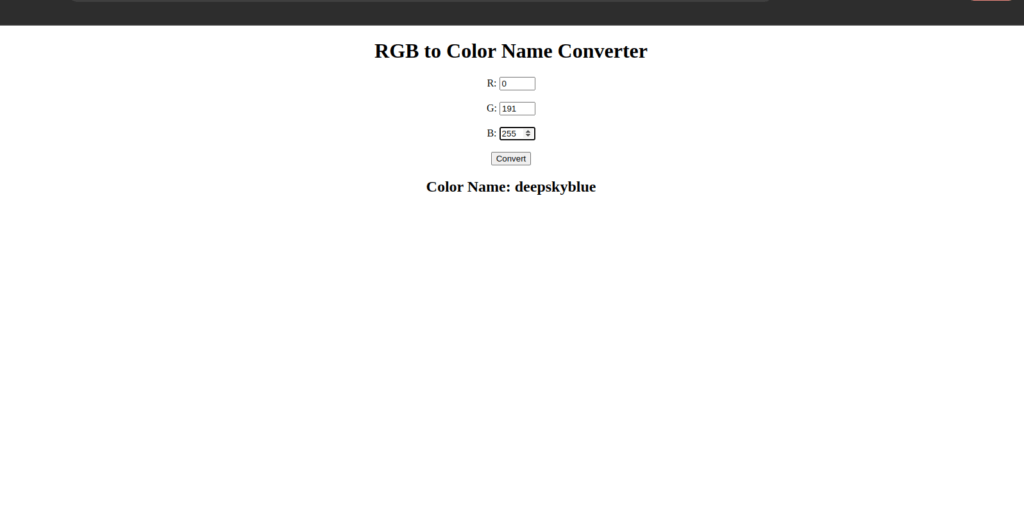
There you have it, Thanks for reading. Happy Coding.