Flask is a microweb framework written using python. You can use it to build web apps. In this tutorial, we will be building a basic word counter.
To begin first install flask. Run the command below to install
pip3 install flask
Now let’s create a folder called word-counter. Inside the folder create a file called app.py.
Inside the word-counter folder create a folder called templates and insert a file called index.html
Inside the app.py file import the following modules.
from flask import Flask, render_template, request
Now let’s initialize our flask app as shown below.
app = Flask(__name__)
Now let’s create a function to handle the calculations.
@app.route('/', methods=['GET', 'POST'])
def word_count():
word_count = 0
if request.method == 'POST':
text = request.form['text']
words = text.split()
word_count = len(words)
return render_template("index.html", word_count=word_count)
The above function receives words from the form and the words are calculated and then rendered on the browser.
Now let’s finish by adding the following at the end of the app.py file.
if __name__ == '__main__':
app.run()
Proceed by adding the following form in the index.html file we created above.
<!doctype html>
<html>
<head>
<title>Flask Word Counter</title>
</head>
<body>
<form method="post">
<textarea name="text"></textarea>
<input type="submit" value="Count Words">
</form>
<p>Word Count: {{ word_count }}</p>
</body>
</html>
The above template asks the user to input the words to be counted and then submitted. Now run the server by using the below command.
python3 app.py
You can also use the command below.
flask run
Now open your browser and enter the below address.
http://127.0.0.1:5000
You should now be able to check out your app as shown below.
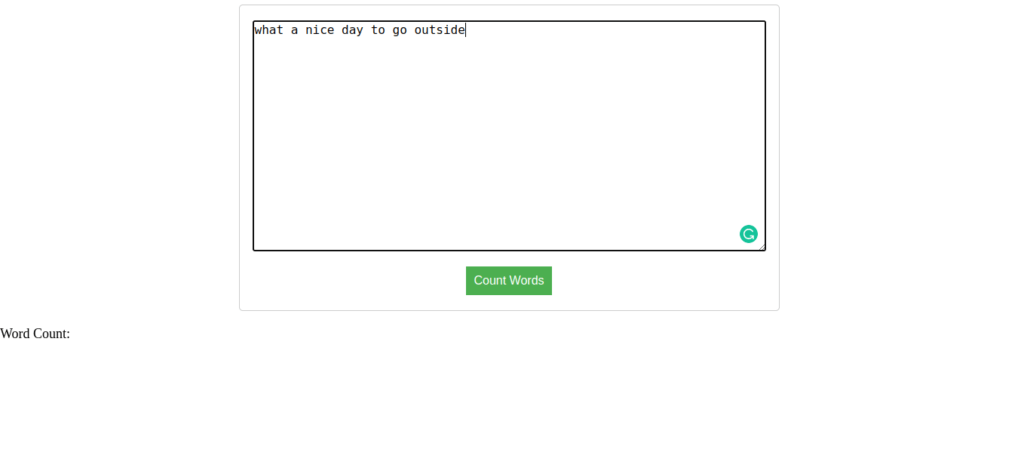
There you have it. Thanks for reading.