Introduction
Rock-paper-scissors is a hand game played between two people, whereby each player simultaneously forms one of three shapes with their hand: a closed fist representing “rock,” an open hand representing “paper,” and a hand with the index and middle fingers extended representing “scissors.”
The objective of the game is to outwit your opponent by choosing a shape that beats theirs. The three shapes have a circular hierarchy where rock beats scissors, scissors beat paper, and paper beats rock. In this tutorial, we will be building a game around the same concept using Python and Django.
Install modules
In this, we will need Django as our web server. to install it run the command below.
pip3 install django
After installing Django let’s now open the terminal and run the command below to create our project.
django-admin startproject django_game
The above command will create a folder called django_game. Cd into the directory and run the command below to create our game folder.
python3 manage.py startapp game
Configure
Now we need to add our app to the django_game project, to do this add the following code in the settings.py file.
INSTALLED_APPS = [
'django.contrib.admin',
'django.contrib.auth',
'django.contrib.contenttypes',
'django.contrib.sessions',
'django.contrib.messages',
'django.contrib.staticfiles',
'game',
]
Now let’s create routes for our project, in the django_game folder inside the urls.py file add the following.
from django.contrib import admin
from django.urls import path,include
urlpatterns = [
path('admin/', admin.site.urls),
path('',include('game.urls')),
]
Game logic
Now let’s create our game logic, all the game mechanics will be handled in the views. Inside the game folder import the following modules.
from django.shortcuts import render
from django.http import HttpResponse
import random
Now let’s create the function below.
def index(request):
options = ['rock', 'paper', 'scissors']
computer_choice = random.choice(options)
player_choice = request.POST.get('choice')
result = None
if player_choice == computer_choice:
result = 'Tie'
elif player_choice == 'rock' and computer_choice == 'scissors':
result = 'You win!'
elif player_choice == 'paper' and computer_choice == 'rock':
result = 'You win!'
elif player_choice == 'scissors' and computer_choice == 'paper':
result = 'You win!'
else:
result = 'You lose.'
return render(request, 'game/index.html', {'computer_choice': computer_choice, 'player_choice': player_choice, 'result': result})
The above code has a list of three items rock, paper, and scissors, the computer uses the list to come up with a random move which is used against the player where the player enters his move in a template and then the move is evaluated in the control flow code above.
Templates
Let’s now create a template to render the game. Inside the game folder create a folder called templates and inside it create a folder called game.
Inside the game folder create a file called index.html. Inside the index.html add the following code.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Rock-Paper-Scissors</title>
<style>
body {
background-color: black;
color: white;
font-family: 'Press Start 2P', cursive;
}
h1 {
font-size: 50px;
text-align: center;
margin-top: 50px;
}
form {
display: flex;
justify-content: center;
margin-top: 50px;
}
input[type="submit"] {
font-size: 30px;
font-weight: bold;
background-color: #FFD700;
border: 5px solid #FFD700;
color: black;
padding: 10px 20px;
margin: 0 10px;
cursor: pointer;
transition: all 0.2s ease-in-out;
}
input[type="submit"]:hover {
background-color: transparent;
color: white;
border-color: white;
box-shadow: 0 0 10px #FFD700;
}
p {
font-size: 30px;
text-align: center;
margin-top: 50px;
}
</style>
</head>
<body>
<h1>Rock-Paper-Scissors</h1>
<form method="POST" action="{% url 'index' %}">
{% csrf_token %}
<input type="submit" name="choice" value="rock">
<input type="submit" name="choice" value="paper">
<input type="submit" name="choice" value="scissors">
</form>
<p>You chose: {{ player_choice }}</p>
<p>Computer chose: {{ computer_choice }}</p>
<p>{{ result }}</p>
</body>
</html>
The above template shows a form where a player will enter his choice and it is sent to the backend for evaluation and the results are rendered below.
Create routes
Inside the game folder create a file called urls.py and add the following.
from django.urls import path
from . import views
urlpatterns = [
path('',views.index, name="index"),
]
Our game should now be ready, open your terminal and run the command below.
python3 manage.py runserver
The above command should start the server which should be accessible at the address shown below in your browser.
http://127.0.0.1:8000
Results
After accessing the address above you should see the game as shown below.
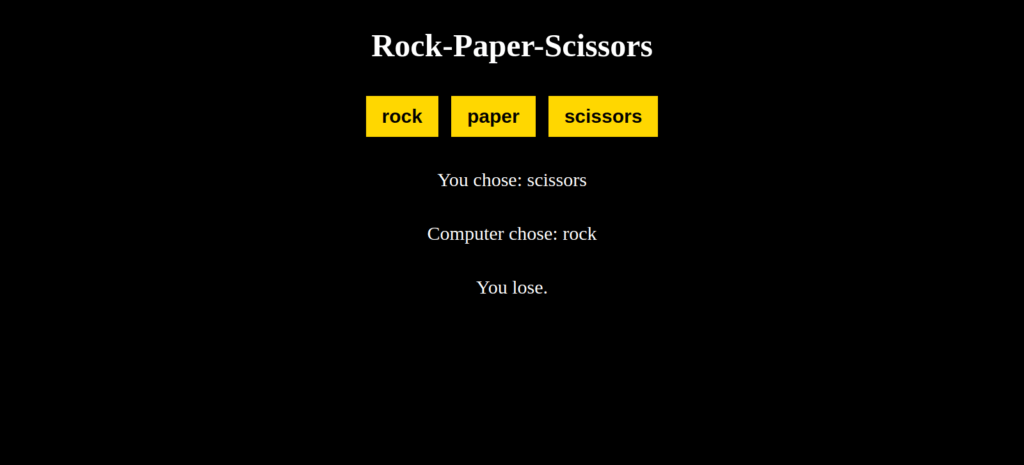
Thanks for reading.