In this tutorial we will be building a simple simple interest calculator app using python, you can use it to calculate simple interest. Simple interest is an interest charge that borrowers pay to lenders for a loan.
To begin let’s first create a file called interest.py , inside the file let’s insert the code below.
class SimpleInterestCalculator:
def __init__(self, principal, rate, time):
self.principal = principal
self.rate = rate
self.time = time
def calculateInterest(self):
interest = (self.principal * self.rate * self.time) / 100
return interest
In the code above we first begin by creating a class and then a function to calculate the interest. Next, we then create a loop that will ask the user for the principle, rate, and time until they choose to quit the program.
while True:
try:
principal = float(input("Enter the principal amount (or 0 to exit): "))
if principal == 0:
break
rate = float(input("Enter the interest rate: "))
time = float(input("Enter the time period (in years): "))
calculator = SimpleInterestCalculator(principal, rate, time)
interest = calculator.calculateInterest()
print("Simple Interest: $",interest)
print()
except ValueError:
print("Invalid input! Enter numeric values only.")
print()
print("Exiting...")
SEE ALSO: How to Test Internet Speed Using Python
Open your terminal and run the command python3 interest.py and you should see the calculator as shown below.
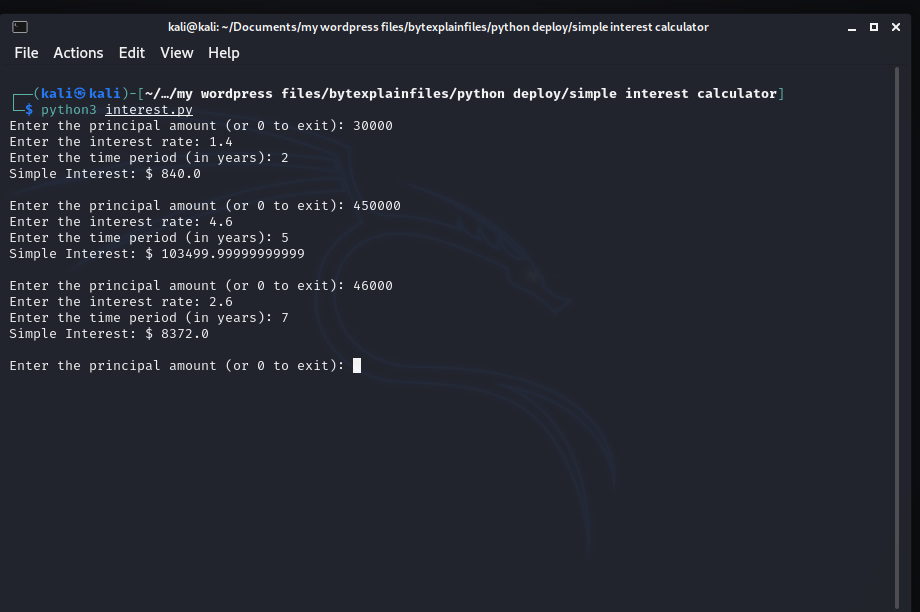
There you have it, Thanks for reading.