Introduction
In this tutorial, we will be building an IP address tracker using Django. With this app, you should see the IP address’s latitude, longitude, country, zip code, and many other details.
Table of Contents
Install modules
To begin we will first need to install Django, to do so run the command below in your terminal.
pip3 install Django
Project setup
To begin we will first need to create our Django project, run the command below to create it.
django-admin startproject django_iplogger
After the command has been executed successfully a folder called django_iplogger will be created, cd into the directory, and then run the command below to create our application.
python3 manage.py startapp iplogger
SEE ALSO: How to build a notes app using Django
Project configuration
Now after the above process is over, let’s add our iplogger application to the django project django_iplogger. Inside the settings.py file in the django_iplogger file add the following code in the installed_apps list.
INSTALLED_APPS = [
'django.contrib.admin',
'django.contrib.auth',
'django.contrib.contenttypes',
'django.contrib.sessions',
'django.contrib.messages',
'django.contrib.staticfiles',
'iplogger',
]
Create IP Address tracking view
Inside the views.py file in the iplogger folder let’s create a view as shown below.
from django.shortcuts import render
import requests
def iptracker(request):
if request.method == 'POST':
ip_address = request.POST['ip_address']
results = get_ip_details(ip_address)
return render(request, 'iplogger/index.html', {'results': results})
else:
return render(request, 'iplogger/index.html')
def get_ip_details(ip_address):
url = f"http://ip-api.com/json/{ip_address}"
response = requests.get(url)
data = response.json()
if data["status"] == "fail":
return "Failed to track IP address"
country = data["country"]
city = data["city"]
zip_code = data["zip"]
latitude = data["lat"]
longitude = data["lon"]
result = f"IP Address: {ip_address}\n"
result += f"Country: {country}\n"
result += f"City: {city}\n"
result += f"Zip Code: {zip_code}\n"
result += f"Latitude: {latitude}\n"
result += f"Longitude: {longitude}\n"
return result
In the above code, we first create a simple function that gets the details of the address from an API and then the results are sent to the template.
Create template
Inside the iplogger let’s create the following files as shown here [ iplogger/templates/iplogger/index.html ] . Now inside the index.html file created let’s create our template as shown below.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>IP Tracker</title>
<style>
body {
background-color: #f1f1f1;
font-family: Arial, sans-serif;
color: #333;
}
.container {
max-width: 400px;
margin: 0 auto;
padding: 20px;
background-color: #fff;
border-radius: 5px;
box-shadow: 0 2px 5px rgba(0, 0, 0, 0.1);
}
h1 {
text-align: center;
margin-bottom: 30px;
color: #555;
}
form {
display: flex;
flex-direction: column;
}
input[type="text"] {
padding: 10px;
margin-bottom: 10px;
border: 1px solid #ccc;
border-radius: 3px;
}
button[type="submit"] {
padding: 10px;
background-color: #4CAF50;
color: #fff;
border: none;
border-radius: 3px;
cursor: pointer;
}
pre {
padding: 20px;
background-color: #eee;
border-radius: 5px;
white-space: pre-wrap;
word-wrap: break-word;
}
</style>
</head>
<body>
<div class="container">
<h1>IP Tracker</h1>
<form method="post">
{% csrf_token %}
<input type="text" name="ip_address" placeholder="Enter IP address">
<button type="submit">Track IP</button>
</form>
{% if results %}
<pre>{{ results }}</pre>
{% endif %}
</div>
</body>
</html>
Create app routes
Inside the iplogger folder create a file called urls.py and then insert the code below. This is where your application routes will reside.
from django.urls import path
from . import views
urlpatterns = [
path('',views.iptracker,name="iptracker"),
]
Create project routes
Finally let’s make our application visible to our project, to do so add the following code in the urls.py file inside the django_iplogger folder.
from django.contrib import admin
from django.urls import path,include
urlpatterns = [
path('admin/', admin.site.urls),
path('',include('iplogger.urls')),
]
Run
Open your terminal and run the command below to start the server.
python3 manage.py runserver
Results
If the command above executes successfully our application should be available at the address [ http://127.0.0.1:8000 ] as shown below.
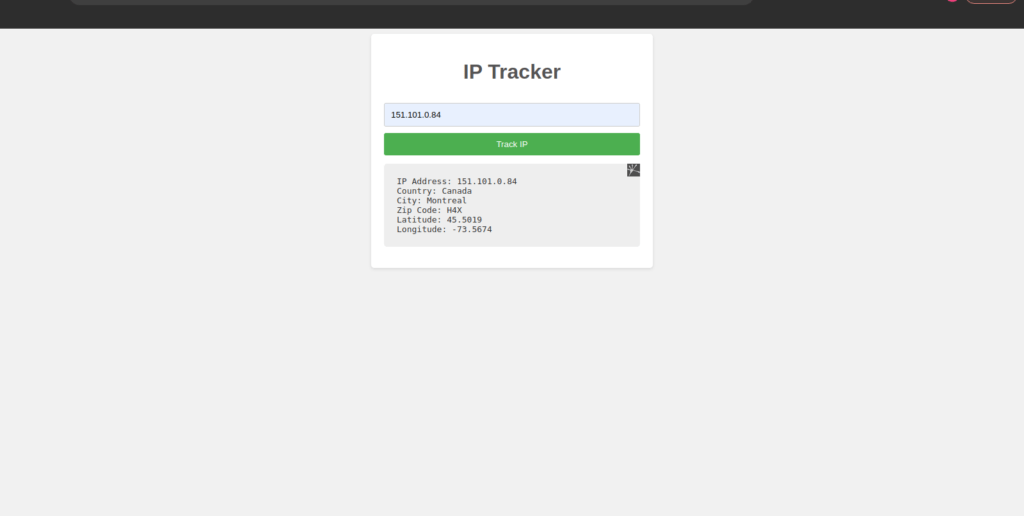
There you have it, Thanks for reading. Happy Coding