In this tutorial, we will be looking at how to convert JSON data to CSV format using Python. To begin we will first need to import the following modules in a file app.py that you will create as shown below.
import json
import csv
Next, we need to create a class that will now convert the JSON data in the file to CSV format as shown below.
class JSON2CSV:
def __init__(self, json_file, csv_file):
self.json_file = json_file
self.csv_file = csv_file
def convert(self):
with open(self.json_file, 'r') as file:
json_data = json.load(file)
with open(self.csv_file, 'w', newline='') as file:
writer = csv.writer(file)
headers = list(json_data[0].keys())
writer.writerow(headers)
for data in json_data:
writer.writerow(data.values())
Now let’s call our class and define the path to our files.
json_file = 'info.json'
csv_file = 'data.csv'
converter = JSON2CSV(json_file, csv_file)
converter.convert()
Now we need to run the command and here is our converted data in a CSV format.
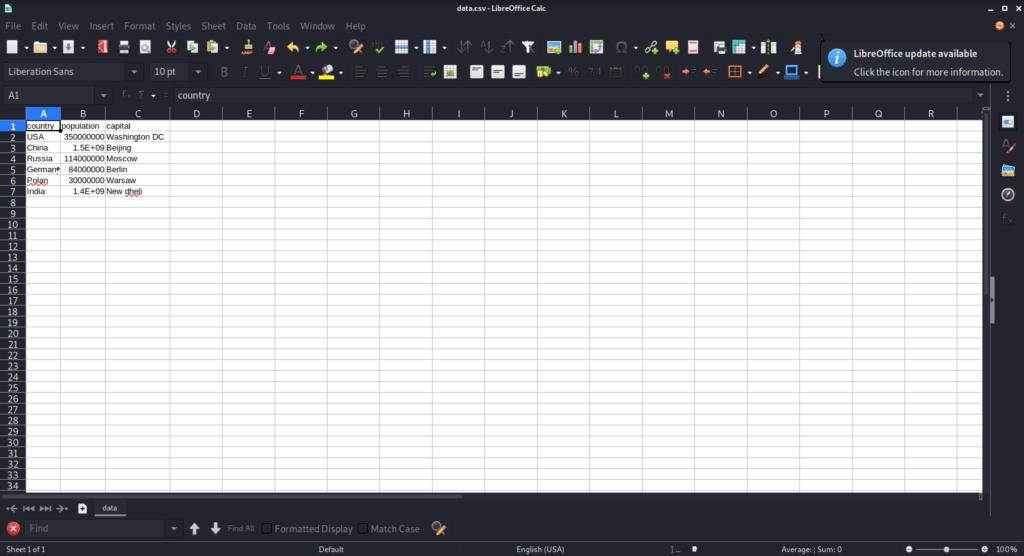
There you have it, Thanks for reading. Happy Coding.