Introduction
Cryptocurrency briefly is digital money that uses cryptography for security and operates independently of central banks or governments. In this tutorial, we will be building a Flask app that analyses different cryptocurrencies in the market.
Table of Contents
Install modules
To begin we will first install a few modules such as Flask which will serve as our primary web server, requests which will be used to make HTTP requests. To install the modules above run the commands shown below on your terminal.
pip3 install FLask
pip3 install requests
File structure
Now let’s create a folder called crypto-app and create a folder called templates with a file index.html in it. Now in the crypto-app folder create a file called app.py which will bear our backend code.
Import modules
Our application will be using the coinstats API to fetch different cryptocurrencies. To begin let’s first import a few modules as shown below.
from flask import Flask , request, render_template
import requests
Next, let’s define a few variables as shown below.
url = 'https://api.coinstats.app/public/v1/coins'
params = {'skip': 0, 'limit':40}
response = requests.get(url, params=params).json()
Above is the URL where the data lie, next we pass the parameters onto our request such as the number of cryptocurrencies we need to get per request Next let’s create a view to display the data to the browser.
app = Flask(__name__)
@app.route('/')
def index():
data = response['coins']
return render_template("index.html",data=data)
if __name__ == "__main__":
app.run(debug=True)
Now let’s create a template to display the data above. In the template we created earlier let’s insert the code below.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<style>
.card {
text-align: center;
}
.crypto {
text-align: center;
}
</style>
<div class="crypto">
<h1>Crypto Statistics</h1>
</div>
<div class="card">
{% for i in data %}
<div>
<h3>{{ i.name}}</h3>
<p>Rank : {{ i.rank}}</p>
<p>Price : {{ i.price}}</p>
<p>Price BTC : {{i.priceBtc}}</p>
<p>Volume : {{i.volume}}</p>
<p>MarketCap : {{ i.marketCap }}</p>
<p>Available Supply : {{i.availableSupply }}</p>
<p>Total Supply : {{ i.totalSupply}}</p>
<p>Price Change 1h : {{ i.priceChange1h }}</p>
<p>Price Change 1d: {{ i.priceChange1d }}</p>
<p>Price Change 1w : {{ i.priceChange1w }}</p>
<a href="{{i.websiteUrl}}">{{i.websiteUrl}}</a>
</div>
{% endfor %}
</div>
</body>
</html>
Run
To run our app, open your terminal and run the command below.
python3 app.py
Results
After running the command above, our application should be available at the address [ http://127.0.0.1:5000 ] on your browser as shown below.
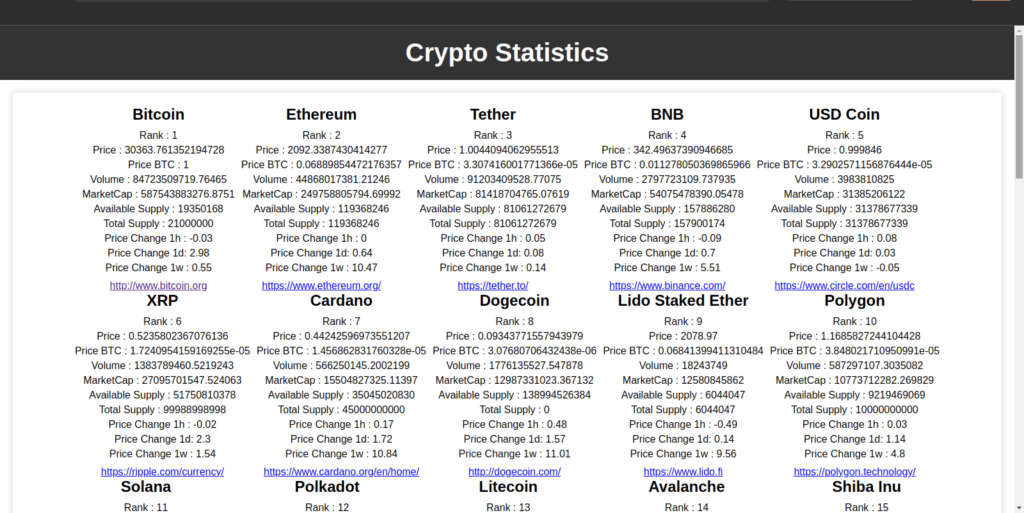
There you have it, thanks for reading. Happy Coding.