In this article, we will be demonstrating how to build a simple audio player using HTML and a bit of JavaScript. You will be able to access various aspects of the audio, such as the audio length, the current timeframe, and many more. Here is the code snippet below.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Enhanced HTML Audio Player</title>
</head>
<body>
<h2>Enhanced Audio Player Example</h2>
<audio id="audioPlayer" controls>
<source src="audio.mp3" type="audio/mp3">
Your browser does not support the audio element.
</audio>
<p id="currentTime">Current Time: 0:00</p>
<p id="duration">Duration: 0:00</p>
<script>
var audioPlayer = document.getElementById('audioPlayer');
var currentTimeElement = document.getElementById('currentTime');
var durationElement = document.getElementById('duration');
// Update the current time and duration
audioPlayer.addEventListener('timeupdate', function() {
var currentTime = formatTime(audioPlayer.currentTime);
var duration = formatTime(audioPlayer.duration);
currentTimeElement.textContent = 'Current Time: ' + currentTime;
durationElement.textContent = 'Duration: ' + duration;
});
// Format time in minutes and seconds
function formatTime(time) {
var minutes = Math.floor(time / 60);
var seconds = Math.floor(time % 60);
seconds = seconds < 10 ? '0' + seconds : seconds;
return minutes + ':' + seconds;
}
</script>
</body>
</html>
Here is the output.
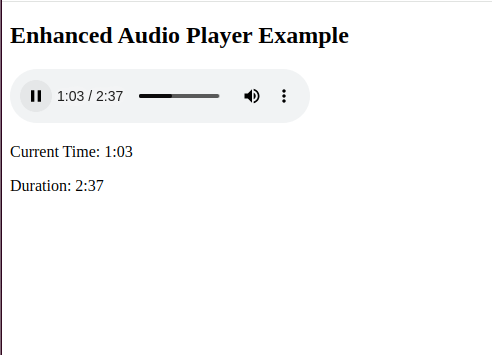
Try it yourself.