A radio button is a graphical control element that allows the user to choose only one of a predefined set of mutually exclusive options. In this article, we will be implementing a basic radio button using HTML. Here is a simple HTML code snippet.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>HTML Radio Buttons Example</title>
</head>
<body>
<h2>Choose a Color:</h2>
<input type="radio" id="red" name="color" value="red">
<label for="red">Red</label>
<input type="radio" id="blue" name="color" value="blue">
<label for="blue">Blue</label>
<input type="radio" id="green" name="color" value="green">
<label for="green">Green</label>
<p>Selected color: <span id="selectedColor"></span></p>
<script>
// Function to update the selected color
function updateSelectedColor() {
var selectedColorElement = document.getElementById('selectedColor');
var selectedColor = document.querySelector('input[name="color"]:checked');
if (selectedColor) {
selectedColorElement.textContent = selectedColor.value;
} else {
selectedColorElement.textContent = 'None';
}
}
// Add event listeners to radio buttons
var radioButtons = document.querySelectorAll('input[name="color"]');
radioButtons.forEach(function(radioButton) {
radioButton.addEventListener('change', updateSelectedColor);
});
</script>
</body>
</html>
Here is the output.
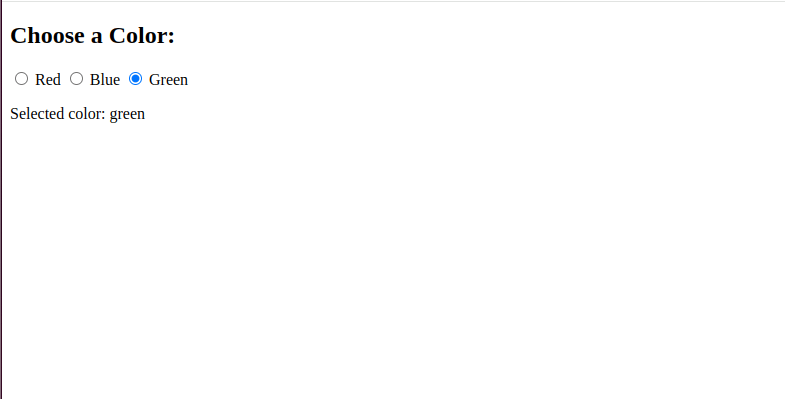
Try it yourself.