In this tutorial, we will be building a simple video player using HTML and a bit of Javascript. Here is a simple code snippet of the video player.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title> HTML Video Player</title>
</head>
<body>
<h2>Video Player</h2>
<video id="videoPlayer" controls width="600">
<source src="earth.mp4" type="video/mp4">
Your browser does not support the video element.
</video>
<p id="currentTime">Current Time: 0:00</p>
<p id="duration">Duration: 0:00</p>
<script>
var videoPlayer = document.getElementById('videoPlayer');
var currentTimeElement = document.getElementById('currentTime');
var durationElement = document.getElementById('duration');
// Update the current time and duration
videoPlayer.addEventListener('timeupdate', function() {
var currentTime = formatTime(videoPlayer.currentTime);
var duration = formatTime(videoPlayer.duration);
currentTimeElement.textContent = 'Current Time: ' + currentTime;
durationElement.textContent = 'Duration: ' + duration;
});
// Format time in minutes and seconds
function formatTime(time) {
var minutes = Math.floor(time / 60);
var seconds = Math.floor(time % 60);
seconds = seconds < 10 ? '0' + seconds : seconds;
return minutes + ':' + seconds;
}
</script>
</body>
</html>
Here is the output.
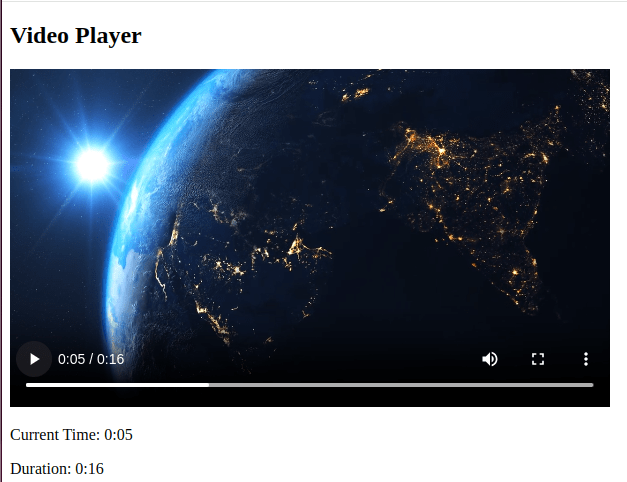
Try it yourself.