Introduction
CherryPy is a lightweight, object-oriented web framework built using python. It is designed to be flexible, simple, and easy to learn, and can be used to build web applications of any size. In this tutorial, we will be building a simple hello world application.
Table of Contents
Install modules
To begin using we first need to install the library into our computer, to do so open your terminal and run the command below.
pip install CherryPy
File structure
After cherrypy is installed let’s create a folder called helloworld , you can name it anything, inside the folder create a file and name it app.py
Import modules
Inside the app.py file let’s import our module as shown below.
import cherrypy
Route
Now let’s create our class which will handle the hello world response, here is the code below.
class Index:
@cherrypy.expose
def index(self):
return "Hello World!"
if __name__ == '__main__':
cherrypy.quickstart(Index())
Run
Now let’s run our application, in your terminal run the command below.
python3 app.py
After running the command above your applicatioion should be available the address [ http://127.0.0.1:8080 ].
Results
Here is our application as shown below.
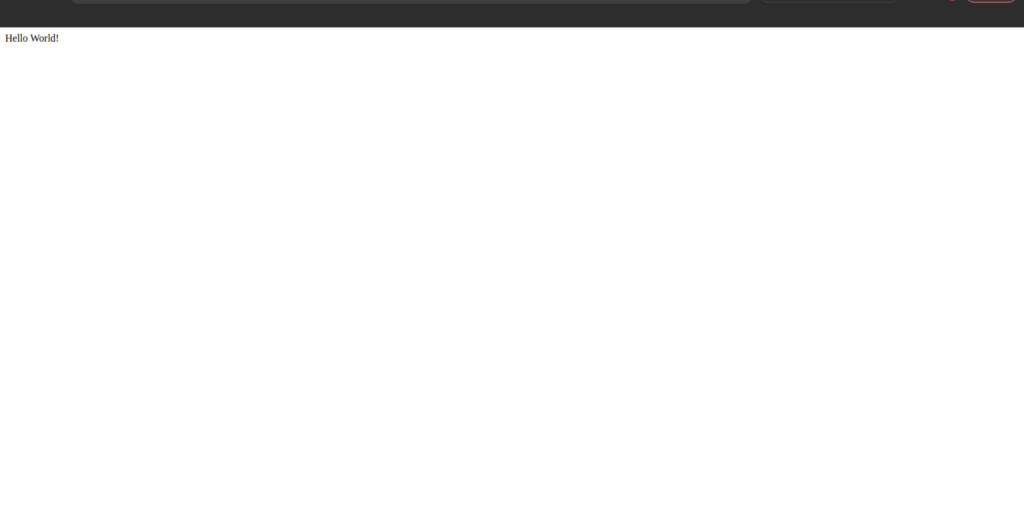
Thanks for reading. Happy coding.