In this tutorial, we will be building a loan calculator app using Flask. To begin we will first need to install Flask by running the command on your terminal.
pip3 install Flask
After installing the above module now let’s create our project file structure as shown below.
loan-calculator
-templates
-index.html
-app.py
Now let’s open the app.py file we have created and import the following modules as shown below.
from flask import Flask, render_template, request
Initialize the application as shown below.
app = Flask(__name__)
Next is our main view, here we will do all the calculations according to the user input as shown here.
@app.route('/', methods=['GET', 'POST'])
def home():
if request.method == 'POST':
principle = float(request.form['principle'])
interest_rate = float(request.form['interest_rate'])
loan_term = float(request.form['loan_term'])
interest = principle * (interest_rate / 100) * loan_term
total_amount = principle + interest
monthly_payment = total_amount / loan_term
return render_template('index.html', principle=principle, interest_rate=interest_rate,
loan_term=loan_term, interest=interest, total_amount=total_amount,
monthly_payment=monthly_payment)
return render_template('index.html')
if __name__ == '__main__':
app.run(debug=True)
Now in the index.html file we created earlier let’s insert the template below.
<!DOCTYPE html>
<html>
<head>
<title>Loan Calculator</title>
<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css">
<style>
.container {
margin-top: 100px;
}
</style>
</head>
<body>
<div class="container text-center">
<h1 class="mb-5">Loan Calculator</h1>
<form action="/" method="POST">
<div class="form-group">
<label for="principle">Principle Amount:</label>
<input type="number" class="form-control" name="principle" value="{{ principle }}" required>
</div>
<div class="form-group">
<label for="interest_rate">Interest Rate:</label>
<input type="number" class="form-control" name="interest_rate" value="{{ interest_rate }}" step="any" required>
</div>
<div class="form-group">
<label for="loan_term">Loan Term (in years):</label>
<input type="number" class="form-control" name="loan_term" value="{{ loan_term }}" required>
</div>
<button type="submit" class="btn btn-primary">Calculate</button>
</form>
{% if principle %}
<div class="mt-5">
<h2 class="mb-4">Loan Calculation Results:</h2>
<p>Principle Amount: {{ principle }}</p>
<p>Interest Rate: {{ interest_rate }}%</p>
<p>Loan Term: {{ loan_term }} years</p>
<p>Interest: {{ interest }}</p>
<p>Total Amount: {{ total_amount }}</p>
<p>Monthly Payment: {{ monthly_payment }}</p>
</div>
{% endif %}
</div>
<script src="https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/js/bootstrap.min.js"></script>
</body>
</html>
Now let’s open our terminal and run the command below.
python3 app.py
Now open your browser at the address [ http://127.0.0.1:5000 ] and here is our app as shown below.
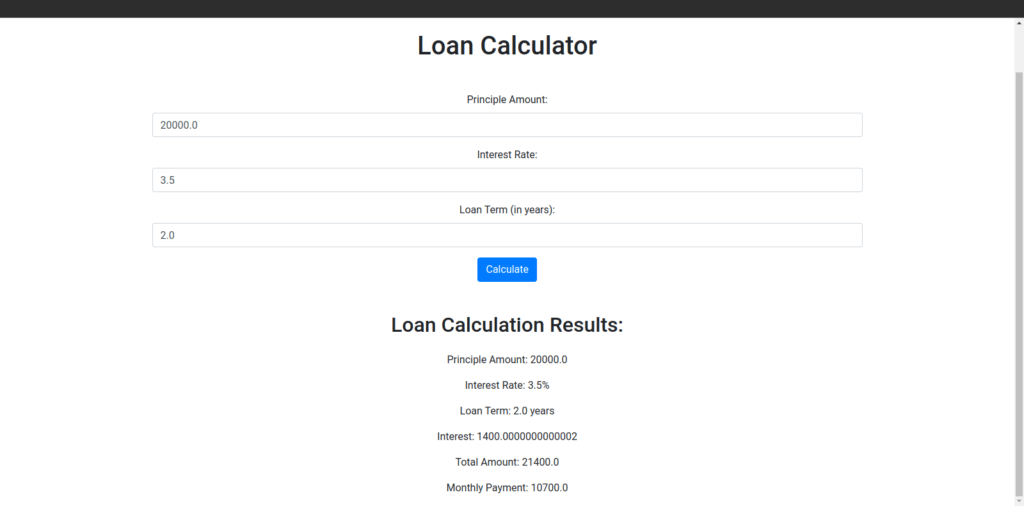
There you have it. Thanks for reading.