Introduction
In this tutorial, we will be looking at how to build a simple API using Flask. To demonstrate we will build a simple password generator where you will add the password length to the end of the URL and a password will be generated according to the given length. For this project, we will be using Flask as our basic web server, flask-restful as our API module .
Install modules
To install the above modules run the commands below on your terminal as shown below.
pip3 install Flask
pip3 install flask-restful
Create app
After installing the above modules create a folder and call it flask-restapi and create a file called app.py. Inside the file import the following modules as shown below.
from flask import Flask
from flask_restful import Resource, Api
import random
import string
Next, let’s initialize our Flask application and the API as shown below.
app = Flask(__name__)
api = Api(app)
Now let’s create a class that generates the password and passes it to the API.
class GeneratePassword(Resource):
def get(self, length=8):
password = ''.join(random.choices(string.ascii_letters + string.digits, k=length))
return {'password': password}
api.add_resource(GeneratePassword, '/password', '/password/<int:length>')
if __name__ == '__main__':
app.run(debug=True)
Run
Open your terminal and run the command shown below.
python3 app.py
Results
Open your browser and open the address [ http://127.0.0.1:5000/password/8 ] and a password should appear as shown below.
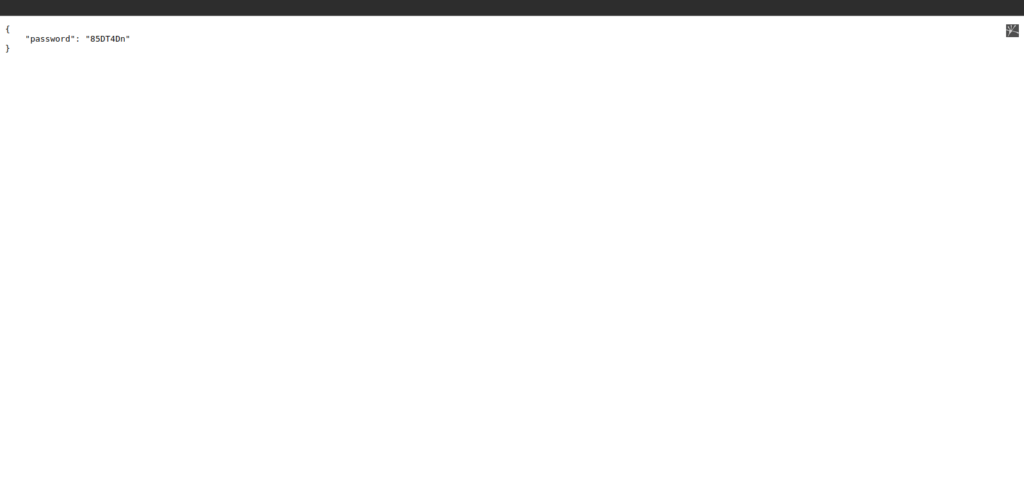
There you have it, Thanks for reading.