Introduction
In this tutorial, we will be building an audio player using howler.js whereby audio files will be served from a Flask server. Howler.js is a JavaScript library that provides a simple and efficient way to play audio in web applications. It offers a range of features for playing and controlling audio files, including playing, pausing, stopping, seeking, volume control, and many more. You can use it to play a wide variety of audio formats.
Table of Contents
Install modules
For serving the audio files for our audio player we will need Flask. To install Flask run the command below in your terminal
pip3 install Flask
File structure
To begin we need to create a folder called flask-howler which will be used to hold our application. Inside the folder create a folder called templates and inside it create a file called index.html. Now back into the flask-howler create a folder called static and add the audio files you want to play.
Now create a file called app.py which will contain code for the backend.
Import modules
Inside the app.py import the modules below.
from flask import Flask, render_template
Create a view
Now let’s create a view to render the template holding the audio controller and add the code below.
app = Flask(__name__)
@app.route('/')
def index():
return render_template('index.html')
if __name__ == '__main__':
app.run(debug=True)
Audio controller template
Now let’s create the audio controller. Inside the index.html template we created above let’s add the code below.
<!doctype html>
<html>
<head>
<title>Flask Audio App</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/howler/2.2.3/howler.min.js"></script>
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css">
<style>
#audio-player {
display: flex;
justify-content: center;
align-items: center;
height: 300px;
}
.heading {
text-align:center;
}
button {
margin: 10px;
}
</style>
</head>
<body>
<h1 class="heading">Flask Audio Player</h1>
<div id="audio-player">
<button id="play" class="btn btn-success btn-lg">Play</button>
<button id="pause" class="btn btn-warning btn-lg">Pause</button>
<button id="stop" class="btn btn-danger btn-lg">Stop</button>
</div>
<script>
var sound = new Howl({
src: ['/static/epic.mp3']
});
document.getElementById('play').addEventListener('click', function() {
sound.play();
});
document.getElementById('pause').addEventListener('click', function() {
sound.pause();
});
document.getElementById('stop').addEventListener('click', function() {
sound.stop();
});
</script>
</body>
</html>
The above code first accesses howler.js using a cdn and then we add a bit of bootstrap styling. Here we have implemented three functionalities that is play, pause, and stop.
Run
Now our app is finished, open your terminal and run the command below.
python3 app.py
After the command above executes successfully our audio player should be accessible on your browser at the address below.
http://127.0.0.1:5000
Results
Here is our audio player. Play around with controls, if you find it it fun you can add more functioalities with reference to howlerjs documentation here.
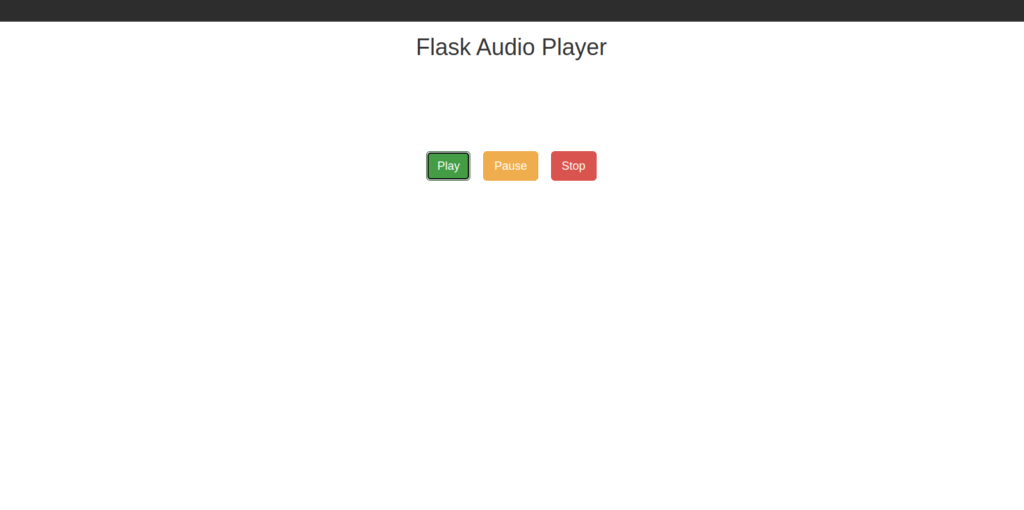
There you have it. Thanks for reading.