Choosing the right backend framework is crucial for developing scalable, secure, and efficient web applications. Backend frameworks provide the necessary tools and libraries to streamline the development process, manage databases, handle user authentication, and more. This blog explores the top 10 best backend frameworks that are dominating the industry in 2024. Whether you’re building a small web application or a large-scale enterprise solution, these frameworks can help you achieve your development goals efficiently.
Table of Contents
1. Django
Django is a high-level Python web framework that encourages rapid development and clean, pragmatic design. Known for its “batteries-included” philosophy, Django provides built-in features such as an ORM, authentication, and a powerful admin panel, allowing developers to focus on building their applications rather than reinventing the wheel.
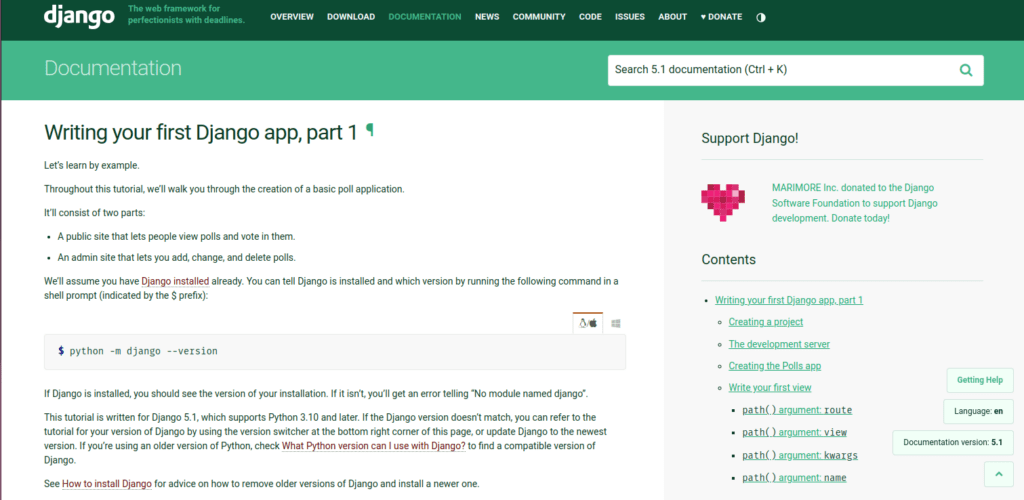
- Pros:
- Rich ecosystem with numerous built-in functionalities.
- Strong security features, such as prevention of SQL injection and cross-site scripting.
- Scalable and versatile, suitable for both small and large applications.
- Cons:
- Steeper learning curve for beginners due to its monolithic nature.
- Can be overkill for simple projects.
Read Also : Top 10 Frontend Frameworks to Elevate Your Web Development Skills in 2024
2. Express.js
Express.js is a fast, unopinionated, and minimalist web framework for Node.js. It provides a robust set of features for building web and mobile applications and is known for its simplicity and flexibility. Express.js is often the first choice for developers building RESTful APIs and server-side applications.
const express = require('express')
const app = express()
const port = 3000
app.get('/', (req, res) => {
res.send('Hello World!')
})
app.listen(port, () => {
console.log(`Example app listening on port ${port}`)
})
- Pros:
- Lightweight and fast, with a minimalistic core.
- Highly flexible, allowing developers to choose their preferred tools and libraries.
- Extensive middleware support for handling requests and responses.
- Cons:
- Lacks built-in features like authentication and ORM, requiring additional configuration.
- Asynchronous nature can be challenging for new developers.
3. Ruby on Rails
Ruby on Rails, or Rails, is a server-side web application framework written in Ruby. It follows the convention over configuration (CoC) and don’t repeat yourself (DRY) principles, promoting efficient and clean coding practices. Rails is known for its simplicity and speed, making it a popular choice for startups and rapid prototyping.
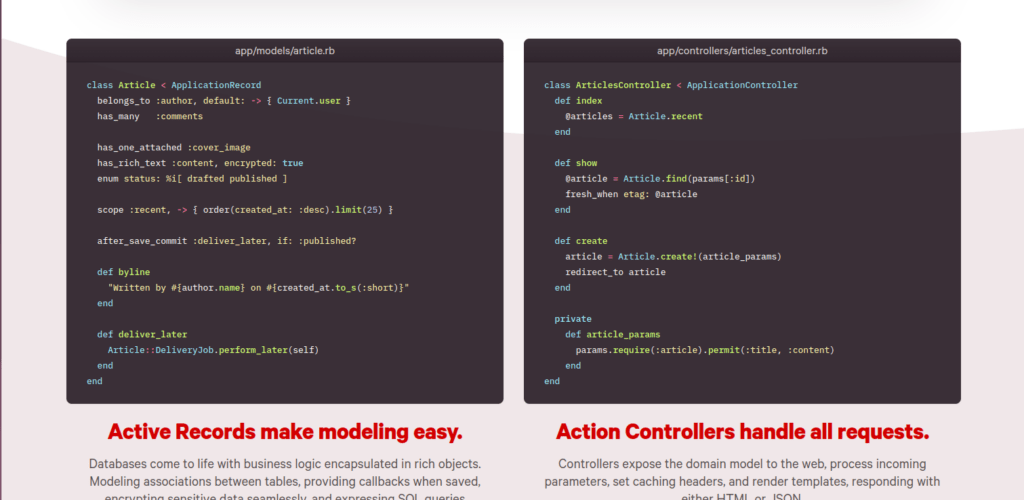
- Pros:
- Rapid development with clear and concise syntax.
- Rich ecosystem with numerous plugins (gems) to extend functionality.
- Strong community support and extensive documentation.
- Cons:
- Performance can be a concern for high-traffic applications.
- Learning curve for those unfamiliar with Ruby.
4. Laravel
Laravel is a PHP framework that simplifies complex tasks like routing, authentication, and caching. With its elegant syntax and comprehensive documentation, Laravel has become a preferred choice for PHP developers. It provides a powerful ORM called Eloquent, built-in tools for unit testing, and an expressive query builder.
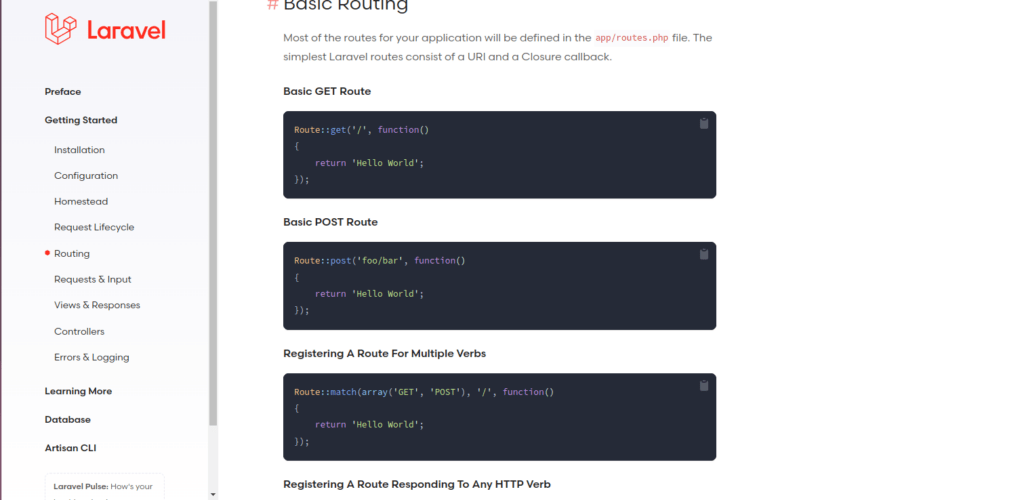
- Pros:
- Easy to learn and use with a clean syntax.
- Extensive ecosystem, including tools for database migration, testing, and security.
- Built-in support for event broadcasting, job queues, and real-time notifications.
- Cons:
- Performance can be slower compared to lightweight frameworks.
- Limited scalability for very large applications.
5. Flask
Flask is a lightweight and flexible Python framework that is ideal for small to medium-sized applications. It follows a modular design, allowing developers to choose the components they need, making it an excellent choice for projects that require customization. Flask’s simplicity and scalability make it popular among both beginners and experienced developers.
from flask import Flask
app = Flask(__name__)
@app.route("/")
def hello_world():
return "<p>Hello, World!</p>"
- Pros:
- Simple and flexible, with a minimalistic core.
- Easy to learn, with straightforward documentation.
- Scalable, allowing for the addition of features as needed.
- Cons:
- Lacks built-in features like authentication and database handling.
- Not as feature-rich as full-stack frameworks like Django.
6. Spring Boot
Spring Boot is an extension of the Spring framework, designed to simplify the development of production-ready applications. It offers pre-configured templates and tools for building scalable, enterprise-level applications with Java. Spring Boot is known for its powerful dependency injection capabilities and extensive ecosystem.
package com.example.demo;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
@SpringBootApplication
@RestController
public class DemoApplication {
public static void main(String[] args) {
SpringApplication.run(DemoApplication.class, args);
}
@GetMapping("/hello")
public String hello(@RequestParam(value = "name", defaultValue = "World") String name) {
return String.format("Hello %s!", name);
}
}
- Pros:
- Robust and mature, with a strong emphasis on scalability.
- Comprehensive documentation and community support.
- Ideal for microservices architecture with built-in support for configuration management.
- Cons:
- Steep learning curve for beginners due to its complex architecture.
- Can be overkill for small applications.
7. ASP.NET Core
ASP.NET Core is a cross-platform, high-performance framework for building modern, cloud-based, and internet-connected applications. Developed by Microsoft, it supports multiple languages and is known for its performance, security, and scalability. ASP.NET Core is ideal for building web applications, microservices, and APIs.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
using Microsoft.AspNetCore.Builder;
using Microsoft.AspNetCore.Hosting;
using Microsoft.AspNetCore.Http;
using Microsoft.Extensions.DependencyInjection;
using Microsoft.Extensions.Logging;
namespace FirstAppDemo {
public class Startup {
// This method gets called by the runtime.
// Use this method to add services to the container.
// For more information on how to configure your application,
// visit http://go.microsoft.com/fwlink/?LinkID=398940
public void ConfigureServices(IServiceCollection services) {
}
// This method gets called by the runtime.
// Use this method to configure the HTTP request pipeline.
public void Configure(IApplicationBuilder app,
IHostingEnvironment env, ILoggerFactory loggerFactory) {
loggerFactory.AddConsole();
if (env.IsDevelopment()){
app.UseDeveloperExceptionPage();
}
app.Run(async (context) => {
await context.Response.WriteAsync(
"Hello World! This ASP.NET Core Application");
});
}
}
}
- Pros:
- High performance and scalability.
- Strong integration with Azure and other Microsoft products.
- Support for cross-platform development.
- Cons:
- Steeper learning curve for developers unfamiliar with .NET.
- Limited community support compared to open-source alternatives.
8. Phoenix
Phoenix is a web framework built on the Elixir programming language. It is designed for high-performance applications and real-time features, such as chat applications and online games. Phoenix leverages the power of Erlang’s VM for scalability and fault tolerance, making it ideal for applications that require low-latency communication.
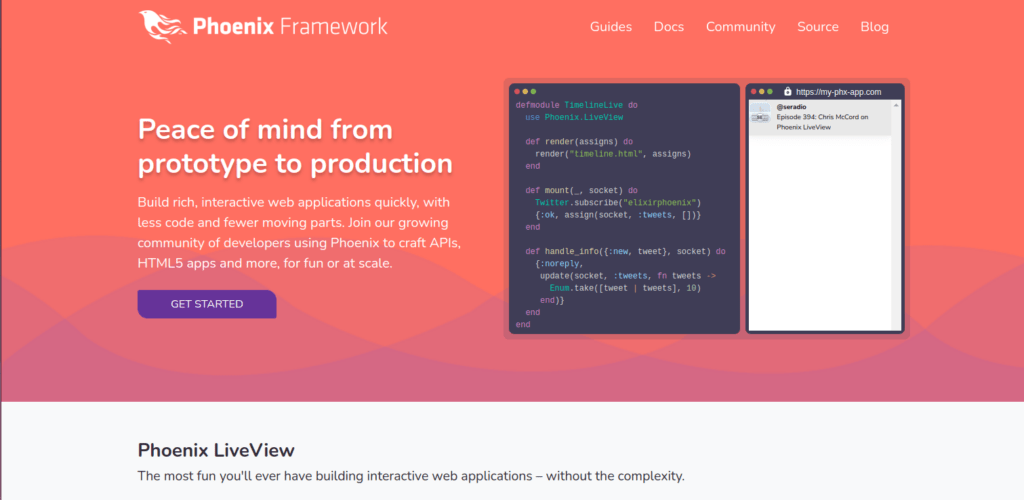
- Pros:
- High performance and real-time capabilities.
- Scalable and fault-tolerant, ideal for high-traffic applications.
- Clean and intuitive syntax, with a focus on developer productivity.
- Cons:
- Smaller community and fewer resources compared to more established frameworks.
- Learning curve for developers new to Elixir.
9. Koa.js
Koa.js, developed by the creators of Express.js, is a lightweight and modular framework for building APIs and web applications. It leverages async functions to simplify error handling and improve readability. Koa’s minimalistic design allows developers to add only the features they need, providing flexibility and control over the application architecture.
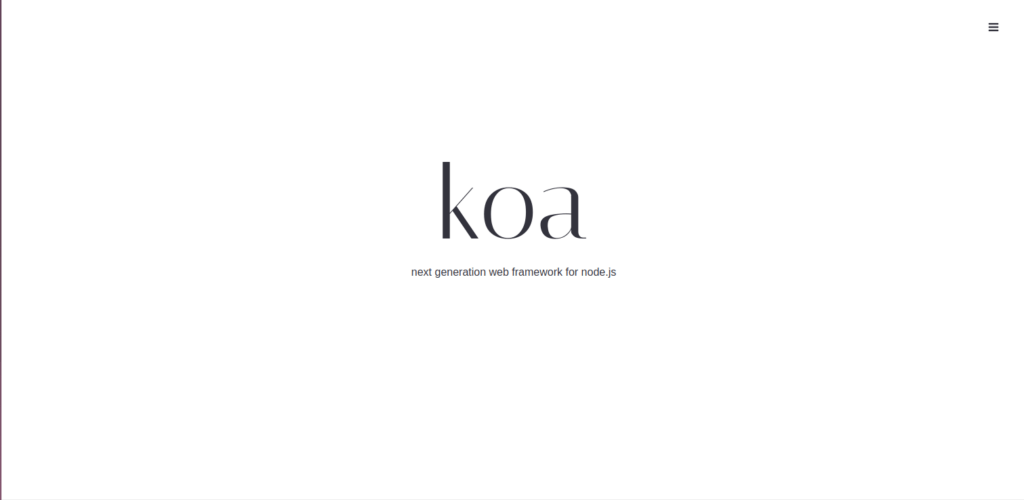
- Pros:
- Lightweight and modular, with a minimalistic core.
- Improved error handling and control flow with async functions.
- Flexible, allowing developers to build from the ground up.
- Cons:
- Requires additional configuration for basic features.
- Smaller community compared to Express.js.
10. NestJS
NestJS is a progressive Node.js framework that is built with TypeScript and inspired by Angular’s architecture. It provides a modular structure, making it ideal for developing scalable server-side applications. NestJS offers a rich set of features out-of-the-box, including support for microservices and GraphQL, making it a powerful choice for enterprise applications.
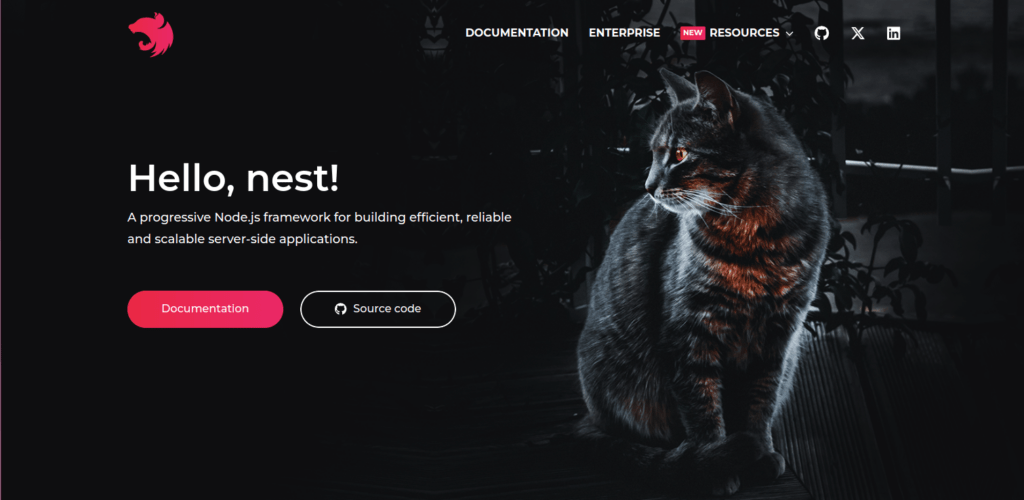
- Pros:
- Modular and scalable, with a strong focus on maintainability.
- Built-in support for microservices and GraphQL.
- TypeScript support for enhanced development experience.
- Cons:
- Steep learning curve for those unfamiliar with Angular or TypeScript.
- Overhead for small projects due to its extensive feature set.
Conclusion
Choosing the right backend framework can greatly influence the success of your web development projects. Each of these frameworks offers unique features and benefits, catering to different project needs and developer preferences. Whether you’re looking for a robust enterprise solution with Spring Boot or a lightweight, flexible framework like Flask, there’s a backend framework that fits your requirements.
Understanding the pros and cons of each framework will help you make an informed decision, ensuring that your application is not only functional but also scalable and maintainable. By mastering these backend frameworks, you can build powerful and efficient applications that stand out in the competitive digital landscape.